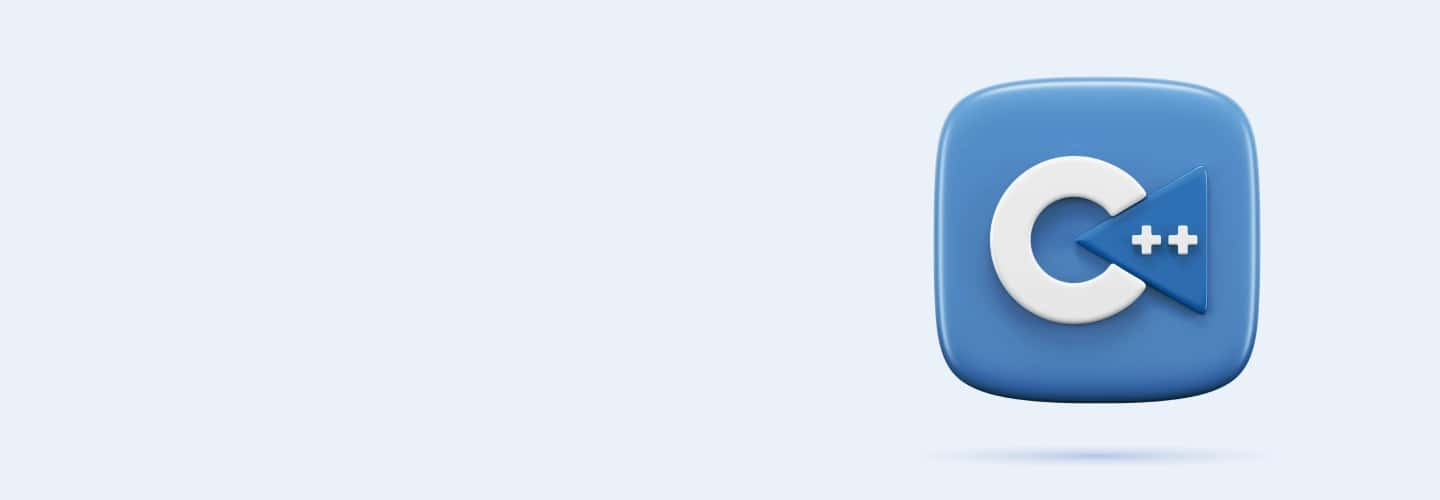
Q1
Q1 What is an object in object-oriented programming?
A code snippet
A variable
An instance of a class
A function
Q2
Q2 Which of the following is a feature of procedure-oriented programming?
Class-based
Focus on functions
Inheritance
Polymorphism
Q3
Q3 In OOP, what does encapsulation refer to?
Storing data in arrays
The process of inheritance
Combining data and methods
A type of loop
Q4
Q4 Which principle of OOP is illustrated by defining different methods with the same name but different parameters?
Inheritance
Polymorphism
Encapsulation
Abstraction
Q5
Q5 What distinguishes a class from an object in OOP?
A class is a blueprint, while an object is an instance of a class
A class cannot contain methods, while an object can
Objects are templates, while classes are real entities
There is no difference
Q6
Q6 Which concept in OOP allows for the same function to be used in different ways based on the object it is associated with?
Encapsulation
Abstraction
Inheritance
Polymorphism
Q7
Q7 Consider the following code snippet:
class Animal { public: void eat() { cout << "Eating..."; } };
What is demonstrated in this code?
Inheritance
Encapsulation
Class definition
Polymorphism
Q8
Q8 Identify the issue in this C++ code snippet:
class Car {
public:
int speed;
void Car() { speed = 0; }
};
Incorrect class constructor syntax
Missing data encapsulation
No error
Inheritance misuse
Q9
Q9 What is the role of comments in a C++ program?
To optimize code execution
To define variables
To provide documentation and explanation
To declare constants
Q10
Q10 Which of these is not a fundamental data type in C++?
int
float
string
char
Q11
Q11 What is a variable in C++?
A constant value
A data type
A storage location with a name
A keyword
Q12
Q12 Which data type would be best to store a person's age?
int
double
bool
char
Q13
Q13 What is a constant in C++?
A variable whose value can change
A fixed value that cannot be altered during execution
A type of operator
A special function
Q14
Q14 What is the output of this C++ code?
int main() {
int x = 5;
cout << x;
return 0;
}
0
5
x
Error
Q15
Q15 Identify the error in this C++ code:
int main() {
int 123number;
return 0;
}
Variable name starting with a digit
Incorrect data type
Syntax error in return statement
No error
Q16
Q16 Spot the error in this C++ code:
const int num; num = 10;
cout << num;
Missing variable initialization
Incorrect use of const
Syntax error in cout statement
No error
Q17
Q17 What is the output of the expression 5 + 3 * 2 in C++?
16
11
10
8
Q18
Q18 Which operator is used for logical AND in C++?
&&
&
||
==
Q19
Q19 What does the ++ operator do in C++?
Divides the value by two
Decreases the value by one
Increases the value by one
Squares the value
Q20
Q20 In C++, what is the difference between == and =?
== is used for assignment, while = is used for comparison
== is used for comparison, while = is used for assignment
Both are used for assignment
Both are used for comparison
Q21
Q21 What is the result of the expression !true && false in C++?
true
false
Syntax error
None of the above
Q22
Q22 What is the output of the following code?
int a = 1;
int b = 2;
cout << (a++ + b);
3
4
Syntax error
2
Q23
Q23 What is the result of int x = 10; x *= 3; in C++?
30
13
33
10
Q24
Q24 Pseudocode:
SET a TO 5 IF a > 3 THEN PRINT "Yes" ELSE PRINT "No" What is the output?
Yes
No
Error
None
Q25
Q25 Pseudocode:
SET x TO 10 SET y TO x MOD 4 PRINT y What does this print?
2
4
10
0
Q26
Q26 Pseudocode:
SET val TO 7 IF val < 10 AND val > 5 THEN PRINT "In range" ELSE PRINT "Out of range" What is the output?
In range
Out of range
Error
None
Q27
Q27 Pseudocode:
SET num TO 15 SET result TO num DIV 2 PRINT result What does this print?
7.5
7
15
Error
Q28
Q28 Pseudocode:
IF (5 > 3) OR (8 < 6) THEN PRINT "True" ELSE PRINT "False" What is the output?
True
False
Error
None
Q29
Q29 Find the mistake:
int num;
num == 5;
cout << num;
Misuse of equality operator
Undeclared variable
No error
Syntax error in cout statement
Q30
Q30 Identify the error:
int x = 10;
int y = ++x + 2;
cout << x << " " << y;
Incorrect use of increment operator
No error
Syntax error in cout statement
Misuse of assignment operator