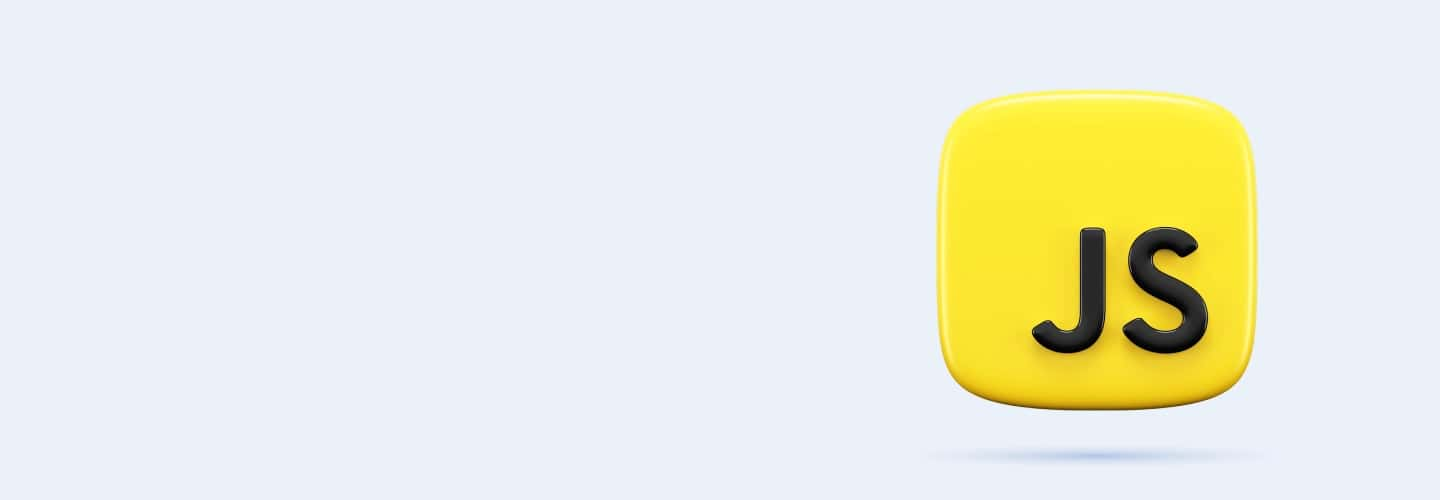
Q1
Q1 Which of the following is a correct syntax to display “Hello World” in an alert box using JavaScript?
alertBox('Hello World');
alert('Hello World');
msgAlert('Hello World');
displayAlert('Hello World');
Q2
Q2 What is the purpose of JavaScript in web development?
To structure web pages
To style web pages
To add interactivity and dynamic content to web pages
To store data on the server
Q3
Q3 Which keyword is used for declaring a variable in JavaScript that can be reassigned?
const
var
let
static
Q4
Q4 In JavaScript, which of the following is a valid variable name?
2names
$name
-name
name2
Q5
Q5 Which data type in JavaScript is used to represent logical values?
String
Boolean
Number
Undefined
Q6
Q6 What does the undefined value in JavaScript represent?
An unassigned variable
A null value
A logical false
An error condition
Q7
Q7 What will be the output of the following code?
console.log(typeof null);
'object'
'null'
'undefined'
'number'
Q8
Q8 Which of the following is an example of a loosely typed language?
Java
C++
JavaScript
Python
Q9
Q9 Which operator is used to check both the value and the type of a variable in JavaScript?
==
===
!=
!==
Q10
Q10 What is the output of the following code snippet?
var a = 10;
console.log(a);
10
'10'
undefined
null
Q11
Q11 What is the output of the following code snippet?
let x = 'Hello';
let y = 'World';
console.log(x + ' ' + y);
HelloWorld
'Hello World'
'Hello' 'World'
Hello World
Q12
Q12 Which statement is used to execute a block of code multiple times in JavaScript?
for
if
return
break
Q13
Q13 What does the if statement in JavaScript do?
Declares a variable
Executes a block of code based on a condition
Prints a message to the console
Loops through a block of code
Q14
Q14 Which of the following is not a loop structure in JavaScript?
while
for
if
do-while
Q15
Q15 In a switch statement, what keyword is used to terminate a case in JavaScript?
end
break
stop
exit
Q16
Q16 What will be the output of the following code?
let a = 2;
if(a > 3) {
console.log('Yes');
} else {
console.log('No');
}
Yes
No
Undefined
Error
Q17
Q17 In a for loop, what are the three optional expressions, separated by semicolons?
Initializer, Condition, Incrementer
Condition, Incrementer, Initializer
Incrementer, Initializer, Condition
Condition, Initializer, Incrementer
Q18
Q18 What is the output of this code snippet?
for (let i = 0; i < 3; i++) {
console.log(i);
}
012
123
0-1-2
1-2-3
Q19
Q19 Consider the following code:
let x = 5;
let result = (x > 3) ? 'Yes' : 'No';
console.log(result);
What is the output?
'Yes'
'No'
true
false
Q20
Q20 Identify the problem in this code:
let i = 0;
while (i < 3) {
console.log(i);
}
Infinite loop
Syntax error
Logical error
No output
Q21
Q21 Find the error in the following code:
for (let i = 0; i <= 5; i++) {
if(i % 2 == 0) continue;
console.log(i);
}
It doesn't print any number
It only prints odd numbers
It only prints even numbers
Syntax error
Q22
Q22 What is the purpose of a function in JavaScript?
To store data
To repeat a task multiple times
To encapsulate code that performs a specific task
To create web pages
Q23
Q23 How do you define a function in JavaScript?
function = myFunc() {}
function: myFunc() {}
function myFunc() {}
myFunc() = function {}
Q24
Q24 What will be the output of this function call?
function sum(a, b) {
return a + b;
}
console.log(sum(3, 4));
3
4
7
Error
Q25
Q25 In JavaScript, what is a callback function?
A function that runs after the page loads
A function passed as an argument to another function
A function that calls itself
A function that performs an HTTP request
Q26
Q26 Which of the following is true about arrow functions in JavaScript?
They do not have their own this context
They can be used as constructors
They must have a return statement
They are the same as traditional functions
Q27
Q27 What is the result of trying to extend the length of an array using a function in JavaScript?
function extendArray(arr) {
arr.push(5);
}
let myArr = [1, 2, 3];
extendArray(myArr);
console.log(myArr.length);
3
4
5
Error
Q28
Q28 Consider the following code snippet:
function greet() {
return 'Hello World';
}
console.log(greet());
What is the output?
'greet'
'Hello World'
undefined
Error
Q29
Q29 What does the following function return?
function checkEven(number) {
return number % 2 === 0;
}
console.log(checkEven(3));
true
false
3
Error
Q30
Q30 Identify the error in this function:
function multiply(a, b) {
console.log(a * b);
}
It does not return any value
It returns the wrong value
Syntax error
No error