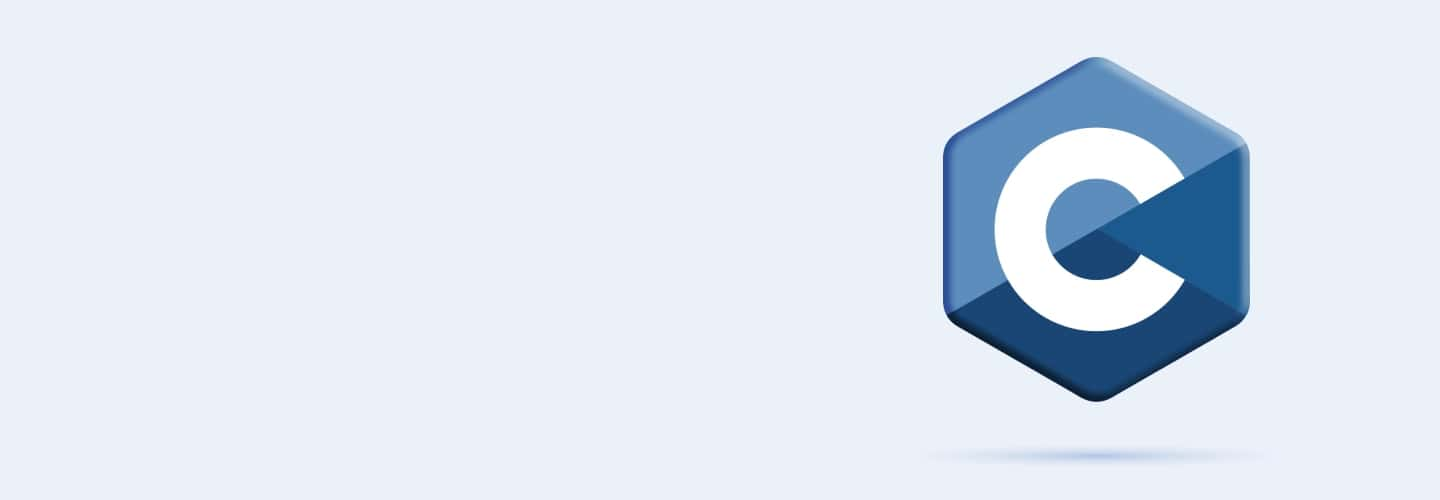
Q1
Q1 Why is learning programming important in today's world?
For academic purposes only
To automate and solve complex problems
Only for software development
For entertainment purposes only
Q2
Q2 What is the main function of a compiler in programming?
To write code
To interpret code
To convert code into machine language
To debug code
Q3
Q3 Which of the following is true about interpreters?
They execute programs faster than compilers
They translate the entire program at once
They translate and execute code line by line
They are not used in modern programming
Q4
Q4 How does a compiler differ from an interpreter?
A compiler executes code, while an interpreter does not
A compiler debugs code, while an interpreter does not
A compiler translates the entire program at once, while an interpreter translates it line by line
A compiler is used only in C programming, while an interpreter is not
Q5
Q5 What is the primary purpose of writing a "Hello World" program when learning a new programming language?
To test if the language supports string operations
To learn complex programming concepts
To demonstrate basic syntax and output
To introduce advanced programming features
Q6
Q6 What will be the output of the following C program?
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
Hello, World!
Syntax Error
0
Nothing
Q7
Q7 Identify the error in this C program:
#include <stdio.h>
int main() {
print("Hello, World!");
return 0;
}
Missing #include statement
Incorrect function name
Syntax error in return statement
No error
Q8
Q8 Which of the following is not a keyword in C?
int
char
include
float
Q9
Q9 What data type would you use to store a character in C?
int
float
char
double
Q10
Q10 Which of the following is a valid identifier in C?
2ndName
_name
#name
none of these
Q11
Q11 What is the size of 'int' data type in C?
Depends on the system
4 bytes
8 bytes
2 bytes
Q12
Q12 What is the difference between 'float' and 'double' data types in C?
Syntax only
Precision
Usage
No difference
Q13
Q13 What will be the output of the following C code?
int main() {
char c = 'A';
printf("%d", c);
return 0;
}
65
A
Syntax Error
None of these
Q14
Q14 Identify the error in this C code:
int main() {
int number = 100.5;
printf("%d", number);
return 0;
}
Assigning float to int
Syntax error
No error
Wrong printf format
Q15
Q15 Spot the error in this code snippet:
#include <stdio.h>
int main() {
const int age; age = 30;
printf("%d", age);
return 0;
}
Uninitialized constant
Missing return statement
Syntax error in printf statement
No error
Q16
Q16 What is the purpose of the printf function in C?
To read input
To print output
To perform calculations
To control program flow
Q17
Q17 What does %d signify in the printf or scanf function?
Double data type
Decimal integer
Dynamic allocation
Directory path
Q18
Q18 What will be the output of the following C code?
int main() {
printf("%d", 500);
return 0;
}
500
%d
Syntax Error
None of these
Q19
Q19 What will the following C code output?
int main() {
int num;
scanf("%d", &num);
printf("%d", num);
return 0;
}
The inputted number
%d
Syntax Error
Nothing
Q20
Q20 Pseudocode:
READ number PRINT "The number is ", number
What does this pseudocode do?
Reads and prints a number
Prints a fixed number
Generates a random number
None of these
Q21
Q21 Identify the error in this C code:
int main() {
int x;
scanf("%d", x);
printf("%d", x);
return 0;
}
Missing & in scanf
Wrong format specifier
No error
Syntax error
Q22
Q22 Find the mistake in this code:
int main() {
float num;
printf("Enter a number: ");
scanf("%f", num);
printf("You entered: %f", num);
return 0;
}
Missing & in scanf
Incorrect format specifier in printf
No error
Syntax error
Q23
Q23 Which operator is used for division in C?
-
*
/
=+
Q24
Q24 What does the '==' operator check?
Assignment
Equality
Greater than
Less than
Q25
Q25 What is the result of the logical expression (1 && 0)?
1
0
True
False
Q26
Q26 What does the '+' operator do in C?
Addition
Subtraction
Multiplication
Division
Q27
Q27 Which operator has higher precedence, '+' or '*'?
*
Both are same
=+
None
Q28
Q28 What does the '!' operator do in C?
Negation
Addition
Multiplication
None
Q29
Q29 What is the output of the expression 2<3?
0
1
2
3
Q30
Q30 What will be the output of the following C code?
int main() {
int a = 10, b = 5;
printf("%d", a / b);
return 0;
}
2
5
0
10